Quick Summary:
Redux vs Context in React state management is an interesting comparison that can be useful for people with questions like ‘can you use Context instead of Redux? Can I mix Context and Redux? Can React Context replace Redux?’. We aim to answer all these questions in this ultimate comparison blog between React Redux and React Context.
React Redux and React Context are great state management solutions in React apps. Complex apps with significant state management needs are more likely to benefit from using the Redux state management approach since it implements discipline and optimizes React performance. For lighter use cases like state passing or theme data passing, the Context API introduced in React is easier since it has been provided out-of-box since React v.16.3.
You can use React Redux and React Context in the same React app for different purposes. You can change some of your React Redux configs to React Context to benefit from its unique offerings and reduce boilerplate code for basic data passing. When to use React Redux vs Context, the better choice for your use case, and how they work are all important questions to discuss. Let’s start with addressing the requirement for React Context and React Redux in the first place.
When to use Redux vs Context vs Prop Drilling for React State Management?
React Redux | React Context | Prop Drilling | |
Purpose | To establish a centralized state management process | To share data between components | Passing data through component tree hierarchy |
State Management Approach | State is centralized in a central store | Decentralized across various contexts (components) | Data passes explicitly from parent to child component |
State Updates | Predictable and controlled | More Frequent | Depends on component hierarchy and flow |
Time Travel Debugging | Supported | No Support | N/A |
Data Flow | Unidirectional data flow | Flexible data flow | Unidirectional data flow |
Learning Curve | Steeper learning curve | Simpler API implementation | Simple |
Boilerplate Code | More boilerplate code | Lesser boilerplate code | Minimal |
Performance Considerations | Performance optimized with memoization | Performance can slow down when overused | Performance can degrade with deeper hierarchies |
Ecosystem | Robust ecosystem with proper middleware and devtools | Basic API configuration and ecosystem | No specific ecosystem |
Primary Use Case | Primarily used for complex data flows | Primarily used for simple data passing | Strict data parsing |
Suitable For | Large and Complex Apps | SMEs | SMEs |
React Context, Redux, and Prop Drilling are all state management approaches in React. However, React Context and Redux are often chosen as preferred alternatives to React Prop Drilling. Let’s check all their use cases and understand when is it better to use which state management approach:
What is Prop Drilling? When to use it?
When the data has to be passed through a higher component to a deeply nested child, every child between that component and the child must accept and pass on the data to its child before reaching the desired child component. This process is known as prop drilling in React.
Prop drilling is the process of passing props from a parent component to the multiple nested child components to make the props data available to that tree’s deeply nested child components.
Prop Drilling can be problematic due to several reasons:
- Creates unnecessary code as you need to pass props through components that have no use for them.
- Any intended or unintended changes in the parent component could break the child component based on the drilled props. Making any changes becomes much more challenging.
- The React app would re-render each component for every component update when props change higher in the three, leading to poor performance issues.
- Components rely on specific props passed down to the tree, making code reusability difficult.
- React app architecture gets overly complex and harder to maintain with deeply nested components, making it harder to scale.
You should use Prop Drilling for State Management only when:
- Your React app is relatively simpler, and the majority of data and functions you need to pass down the component tree are only a few levels deep – ideally
- three or fewer.
- When a few components need data or functions, the prop passing doesn’t cause complexity in the code structure.
What is Redux React? When should you use it?
Redux is a powerful JS library that provides a centralized state management approach for managing data flows in React applications. It can also be used with Angular or Vue.js, though it is popularly paired with React.
Use Redux React for State Management when:
- Your application has complex state management requirements, like having a global state shared and modified by various components.
- Managing the state centrally is crucial.
- You want to leverage its powerful features like time-travel debugging, middleware & predictable state updates.
Benefits of React Redux:
React Redux has been a popular choice amongst top React State Management Tools for Enterprise Applications. Here are some of the prime benefits of using React with Redux for your project:
- Predictable State Management: Redux enforces unidirectional data flow, which makes understanding the app’s state changes easier, leading to more effective debugging and troubleshooting.
- Centralized State: Redux uses a single centralized store for a React application’s state. This enforces the Single Source of Truth concept, which avoids conflicts or chaos in code management.
- Time Travel Debugging: Redux is an effective ecosystem with many tools like Redux DevTools, which allows developers to inspect and travel back in time to debug state changes.
- Scalability: Redux can handle large and complex state management requirements for enterprise application solutions.
- Component Decoupling: With Redux, you can decouple your components from the app’s state. All components can access any state they need through the mapToProps function.
- Testing: Redux makes writing unit tests for actions and reducers easier, leading to a more bug-free codebase.
What is React Context API? When should you use it?
React Context API was introduced in React 16.3, which allows developers to pass data from component trees with the ability to communicate and share data at different levels without having to go through each child component manually. It is known as React ‘Context’ since each component under this principle is ‘context-aware’. Instead of passing down all components through the React component tree, the components needing the particular prop can call or ask for it without relying on intermediaries.
Use React Context API for State Management when:
- Multiple data/functions need to be accessed by various components at different levels of the component tree.
- To avoid excessive prop drilling, keep the component tree readable and maintainable.
- You don’t want to use a full statement management library like Redux for a global application state with many components dependent on it.
Benefits of React Context API:
There are many benefits of using React Context API for handling basic data passing requirements and establishing an effective state management solution:
- Global State Management: React Context allows developers to create and manage a global state that can be shared across multiple components without passing props to intermediate components.
- Prop Drilling Reduction: Prop drilling with deeply nested components has severe performance overheads; using context API can reduce unnecessary code and keep the codebase readable and maintainable.
- Decoupling Components: You can now decouple components from their parent-child relationships, which increases the scope of code reusability and maintainability.
- Support for various data formats: React Context allows you to put any data in the ‘value’ that needs to be shared across various components.
- Multiple Contexts: You can provide multiple context providers for different aspects of the state. This enables the Separation of Concerns and keeps the architecture clean and manageable.
- React Context API Documentation: You can read more about React Context Hooks in React’s official documentation – Built-in React Hooks, which helps manage and resolve any concerns regarding it since it’s an official integration provided by the React team.
Also Read: React Hooks vs Redux
How to Set Up – React Context vs Redux
Now that we have a basic understanding of the use cases and benefits of Redux and Context state management approaches let’s compare them based on ease of setting up. React Context is available as an in-built API from React 16.3. Hence, it requires minimum configuration. In contrast, React Redux is a third-party library that needs to be properly implemented in your React project.
How to setup React Context?
Let’s have a look at the basic step-by-step process of implementing React Context:
1. Create the ‘Context’
You can create the ‘Context’ using React’s ‘createContext’ function. This would return an object with the ‘Provider’ and ‘Consumer’ components.
// MyContext.js
import React, { createContext, useContext } from 'react';
const MyContext = createContext();
export const useMyContext = () ==> {
return useContext(MyContext);
};
export default MyContext;
In this example, we created a custom React hook, ‘useMyContext
’.
2. Provide the Context
Wrap the part of the application component tree where you want the Context to be available with the ‘Provider’ component. This component will accept a ‘value’ prop, which can hold any value in the form of data or function that needs to be shared.
// App.js
import React from 'react';
import MyContext from './MyContext'; // Import the context
const App = () => {
const contextData = {
// Define your context data here
someValue: 'Hello from context!',
someFunction: () => {
// Define your Context functions here
},
};
return (
<MyContext.Provider value={contextData}>
{/* Your application components */}
</MyContext.Provider>
);
};
export default App;
3. Consume the Context
For components needing access to the context data/functions, you need to use the ‘useContext
‘ hook or the ‘Consumer’ component.
Using the ‘useContext’ hook:
// SomeComponent.js
import React from 'react';
import { useMyContext } from './MyContext'; // Import the custom hook
const SomeComponent = () => {
const { someValue, someFunction } = useMyContext(); // Access context data and functions
return (
<div>
<p>{someValue}</p>
<button onClick={someFunction}>Click me</button>
</div>
);
};
export default SomeComponent;
Using the ‘Consumer’ Component
// SomeComponent.js
import React from 'react';
import MyContext from './MyContext'; // Import the context
const SomeComponent = () => {
return (
<MyContext.Consumer>
{(context) => {
const { someValue, someFunction } = context; // Access context data and functions
return (
<div>
<p>{someValue}</p>
<button onClick={someFunction}>Click me</button>
</div>
);
}}
</MyContext.Consumer>
);
};
export default SomeComponent;
Note: Route names must always be distinct.
For more detailed information visit the Official Documentation.
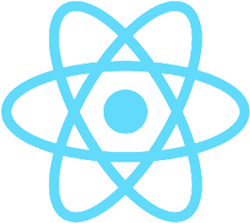
Hola!✋
Are you looking to hire React Developers?
Unleash the boundless potential of your React projects with our elite team of expert React Developers @ Aglowid.
How to setup React Redux?
React Redux is a third-party library, and hence, the implementation process is much more complex and lengthier than compared to React Context. You must install the necessary dependencies and create major React Redux components like Actions, Reducers and linking components to the Redux’s centralized store. Here is a step-by-step guide on installing React Redux:
1. Install all the dependencies
Make sure you have all the dependencies needed in your project. You need:
- ‘react’: you need to download the desired version of React
- ‘redux’: the Redux library
- ‘react-redux’: official binding needed for binding React with Redux.
Install these dependencies using npm or yarn ->
npm install react-redux react-redux
# or
yarn add react redux react-redux
2. Create the Redux Store
Next, you will set up your Redux store and save it in a separate file, naming it ‘store.js
‘ or something similar. Import your Redux setup to this store with any reducers and middleware you need.
// store.js
import { createStore, applyMiddleware } from 'redux';
import rootReducer from './reducers'; // Import the root reducer
import thunk from 'redux-thunk'; // Example middleware, you can add more if required
const middleware = [thunk];
const store = createStore(
rootReducer,
applyMiddleware(...middleware)
);
export default store;
3. Define the Actions and Reducers
Create action creators and reducers for the React application. Actions will describe what should happen, and Reducers will specify how the state needs to change by the corresponding Actions. Create separate files for them – ‘action.js
’ and ‘reducer.js
’.
// actions.js
export const incrementCounter = () => ({
type: 'INCREMENT',
});
// reducers.js
const initialState = { count: 0 };
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
export default rootReducer;
4. Create a Root Component
Wrap the entire application in the Root component with the Provider component from React-Redux. This will help your React app access the Redux store for all its components –
// App.js
import React from 'react';
import { Provider } from 'react-redux';
import store from './store'; // Import the Redux store
import Counter from './Counter'; // Your component that will access the store
function App() {
return (
<Provider store={store}>
<div className="App">
<Counter />
</div>
</Provider>
);
}
export default App;
5. Link Components to Redux
Use the ‘react-redux’ ‘connect’ function to connect a component to the Redux store. This function works on two arguments – mapStatetoProps
and mapDispatchtoProps
. This returns a HoC that can be used to wrap your component.
// Counter.js
import React from 'react';
import { connect } from 'react-redux';
const Counter = ({ count, incrementCounter }) => {
return (
<div>
<p>Count: {count}</p>
<button onClick={incrementCounter}>Increment</button>
</div>
);
};
const mapStateToProps = (state) => ({
count: state.count,
});
const mapDispatchToProps = {
incrementCounter,
};
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
6. Dispatch Actions
In your connected component, dispatch actions by calling the action creators provided by ‘mapDispatchToProps
’. These actions update the state in the Redux store.
7. Access State
Access the Redux Store’s state in any connected component using props mapped to the ‘mapStateToProps
’.
What is the main concept of React Redux – How does it work?
React Redux is a centralized state management approach that manages the state of a React application, providing maximum control in a centralized manner. It follows the Flux architecture pattern principles used for building responsive and performant user interfaces. Facebook developed the Flux Architecture for handling data flow in their web application. Redux is the most popular implementation of the Flux architecture for React, although it also fits well with Angular and Vue.js.
Key Principles of Redux React Architecture:
These are the key principles followed in the Flux architecture that also stand true for React with Redux state management approach:
1. Unidirectional Data Flow:
Data flows in a single direction, from the app’s data store (state) through actions to the views (UI components). This is to maintain predictability and simplify debugging React applications.
2. Actions:
Actions are events that are sent to the Redux Store. They can be triggered by user interactions or called by the React app. All actions have their unique “types” and “payloads”.
3. Dispatcher:
The dispatcher is responsible for distributing the right action to the right Reducers. Think of it as a central hub that manages the data flow in your React application.
4. Stores:
The Redux store is where the app’s logic and state are held for handling actions. Stores are implemented through Reducers.
5. Reducers:
Responsible for taking the current state and returning the changed state per the received relevant action.
6. Views:
The React Components are like view in this Flux-based architecture pattern. They receive information via the stores and display it to the end user. Once a user interacts or performs an action on the UI, components update the state according to those actions.
How does React Context work internally?
React Context is an API integration feature that allows React developers to manage global states without passing props through multiple levels of components. It’s a much more effective way to share data and state across your component tree. Most developers choose React Context to deal with their prop drilling issues, which leads to additional code and performance issues.
Key Principles of React Context Architecture:
These are the key principles that go behind the architecture and functioning of the React Context API for smoother state management:
1. Context Creation:
You start by creating a ‘React.createContext()
’ context. This function returns the object with a ‘Provider’ and ‘Consumer’ components.
2. Provider Component:
When you use the ‘Provider’ component, you wrap a part of the component tree that accepts the ‘value’ prop.
3. Value Prop:
The ‘Value’ Prop contains the data that needs to be shared with various components (Consumers) at different component levels in the component tree. It can hold any value like objects, functions or even the state itself.
4. Consumer Component:
Consumers are the components that want access to the ‘value’ provided by the ‘Provider’ component. You can use React useContext
hook or the ‘Consumer’ component for this. They would automatically subscribe to any changes made in the context data.
5. Context Tree:
React manages a ‘context tree’ as a programming language, similar to the standard component tree. Each ‘Provider’ is assigned to a node in this tree. When the Provider’s ‘value’ prop changes, it updates the ‘context tree’ with the updated ‘value’.
6. Propagating Changes:
To reflect all the changes made in any component, React re-renders the components within the ‘Provider’ subtree whenever the context value changes. Components that use the React useContext or Consumer hook would be re-rendered, too, when the context value changes.
7. Re-rendering Optimization:
Imagine if the React app would re-render the entire application for the smallest changes in any context. If your project is enterprise-level and has a complex architecture with various components, this could affect performance. Luckily, React has a workaround technique to this known as ‘Tree Reconciliation’, which only updates the components affected by the changes.
8. Multiple Contexts:
React Context allows you to set up multiple context providers in your application. The ‘Consumer’ always binds or matches with the right ‘Provider’ by matching them to the nearest one in the tree.
9. Default Values:
React Context provides a default value when creating a context by using ‘React.createContext(defaultValue)
for situations where no ‘Provider’ is found in the component tree.
When to Choose What: React Context vs Redux?
React Context and Redux work towards improving your React apps’ overall state management efficiency. However, Redux is a more comprehensive solution for handling complex tasks, whereas Context is primarily used for establishing efficient data passing between components and has a niche use case.
If you are still confused about when to use what, here is a straightforward guide to help you choose between Redux and Context API:
Use Redux over Context API for when:
- Complex Global State: Redux is better suited for enterprise applications with complex global states. Applications that have large chunks of shared data, deeply nested components or confusing data flows can benefit from Redux’s unidirectional flow and other strict rules to gain some clarity on the project’s architecture.
- Large and Complex Applications: Redux is generally used in large applications such as ecommerce solutions, content management systems or apps with complex business logic.
- Team Familiarity: Most React Development Teams are familiar with React-Redux, which might make it an easier choice to continue using it, especially if you already have a Redux-based codebase.
Use Context API over Redux when:
- Quicker Set-Up: React Redux is extremely lightweight and has an integrated API approach to state management, which makes it easy to set up, too.
- Component-Level State: If your state management needs are at the component level or only need a few components to share data, React Context can easily perform such tasks without worrying about Redux’s overhead.
- Flexibility: If you don’t prefer the strict rules implemented by Redux, you would prefer React Context, as it doesn’t impose strict patterns or conventions.
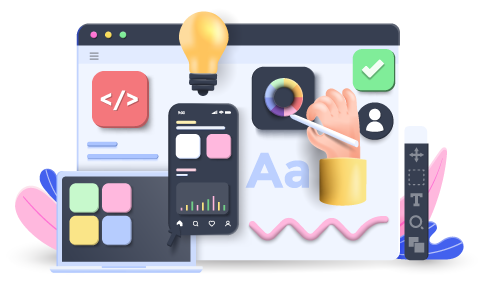
Empower Your Digital Vision with Our Skilled Web Development Team @ Aglowid
Wrapping Up!
This is your ultimate guide on Context vs Redux state management approaches for your React application. Whether you use React with Redux or the Context API via Hooks, the implementation process and best practices need to be followed carefully for a successful implementation. Remember to understand your project requirements, performance tradeoffs you are willing to make and scalability requirements before choosing between React Context and React Redux.
FAQs
React Redux is a centralized state management solution focusing on overall state management solutions. In contrast, Context Hooks are mainly used for optimizing data passing requirements and avoiding excessive prop drilling.
The major differences between React Redux and Context Hooks are:
✦ Library vs Core React – React Redux is a library built for state management in React, whereas React Context is built-in, the official React built after version 16.3.
✦ Learning Curve – Redux has concepts like Reducers, Actions, and Middleware, which can create more of a learning curve for new developers. On the other hand, React Context uses core React concepts like Context and Hooks, which are much easier for React developers.
✦ Centralized vs Decentralized State – React Redux follows a centralized state management approach through a single centralized store. In contrast, Context can be used for centralized and decentralized states, depending on how you structure your Context.
✦ Customization and Flexibility – React Component provides more customization and flexibility options for designing state management solutions, whereas Redux has a structured opinionated pattern.
✦ Primary Use Case – React Redux is primarily an ideal choice for overall state management of complex apps, whereas React Context is better for basic data passing requirements.
React Context can be used as an alternative to Redux, especially for simpler apps with low state management complexity.
Ideal use cases where replacing Redux with Context can be feasible are:
✦ Global UI Themes such as light mode/dark mode
✦ Authentication and User Data without multi-layered role-based permissions
✦ Language Localization
✦ Simple Caching
Yes, Context and Redux can be mixed into a single React application to benefit from their features and capabilities. However, it is not a common practice to do so. If you are still curious about using both in your project…
Here are some of the ways to use Context with Redux in React Apps:
✦ Isolated Components: Use React Context for managing the state with the isolated components where the state is simple and doesn't need to be shared globally. For complex components, continue using Redux.
✦ Iterative Migration: If you plan to migrate from one state management approach to another, Redux to Context or vice versa, you can gradually refactor your components and phase out either one when it's no longer needed.
✦ Redux for Core React State: You can use Redux for core state management and start using Context for less critical component-specific states.
✦ Selective Integration: In some situations, prop drilling or Context passing is difficult for a deeply nested component. You can create a Redux container for that particular component and connect it to the centralized Redux store.
It is still better practice to use one-state management throughout your React app; however, if you are dedicated to using React Context and Redux together, do it through a professional React.js development company.
As a complete override of Redux from being one of the most popular state management approaches no, React Context cannot replace Redux. However, SMEs who don't want to deal with the complexities of Redux can replace it with React Context for sharing state between two components with reduced dependencies.