Quick Summary:
Sick of messy code in you react projects? Wondering how to keep those things neat without extra divs? Enter – React Fragments! This blog is your guide to cleaner code and simpler structure. With easy syntax, benefits, and giving real-life examples. No more clutter, just react development made simple. Dive in & transform your react coding game.
React is the popular JavaScript library for building user interfaces, has made it easier for developers to create dynamic and responsive web applications. However, one challenge that developers often face is the requirement to return a single root element from a component’s render method. This limitation can lead to unnecessary nesting and cluttered markup, making the code more difficult to read and maintain.
Enter React Fragments, a feature introduced in React 16.2 that provides a clean and efficient solution to this problem. Fragments allow developers to group a list of child elements without adding an extra node to the Document Object Model (DOM), resulting in cleaner and more semantic HTML. This guide will delve into the power of React Fragments, exploring their syntax, benefits, use cases, and best practices for incorporating them into your React applications.
What is React Fragment?
React fragment was introduced in 16.2.0. It is a feature provided by the react that provides a way to group multiple elements without introducing an additional parent node in DOM. In traditional React components, returning multiple elements requires them to be wrapped in the container element or div. However, this approach could lead to unnecessary and non-semantic HTML structure.
React Fragments address this issue by allowing you to group elements without creating an extra DOM node. They can be implemented using either empty angle brackets (<> </>) or the <React.Fragment> syntax. This feature improves code readability, maintains a cleaner DOM structure, and enhances the overall development experience by avoiding unnecessary wrapper elements. React Fragments are particularly useful when rendering lists, tables, or in scenarios where maintaining a clean and semantic HTML structure is essential. Checkout the official documentation here.
Syntax
function Test(){
return (
<div>
<h1> Hello! This is Heading 1</h1>
<p> Hey! This is tag is used to write paragraphs </p>
<h2> Hi! This is Heading 2 </h2>
</div>
);
};
export default Test;
Well this is structure is fine, it may cause the unintended issues in our components. Therefore, to avoid having such issues, we are going to use React Fragments here.
function Test() {
return (
<React.Fragment>
<h1> Hello! This is Heading 1</h1>
<p> Hey! This is tag is used to write paragraphs</p>
<h2>Hi! This is Heading 2</h2>
</React.Fragment>
);
}
}
Apart from this there is also another way known as shorter syntax, we can use for declaring React Fragments. This is by far the easiest and the cleanest way to use React Fragments. It almost feels like you’re using the regular HTML elements.
function Test() {
return (
<>
<h1> Hello! This is Heading 1</h1>
<p> Hey! This is tag is used to write paragraphs</p>
<h2>Hi! This is Heading 2</h2></>
);
}
}
What are the Benefits of Using React Fragment?
Every node of the DOM Tree is important in React Development. React. Fragments is the unsung hero, offering not only cleaner codes but also substantial React performance optimization advantages. React Fragments is a game changer. Let’s explore the benefits.
Efficient DOM Structure
The traditional approach of using div wrappers leads to an unnecessarily increased depth in the DOM tree. React Fragments saves nodes because they eliminate the need for extra divs. The efficiency of this technique increases as the depth and complexity of the tree increase, leading to significant rendering savings.
Improved Performance
React Fragments reduce memory consumption and render faster by reducing the number of nodes within the DOM. These performance gains are crucial in scenarios where complex applications require many DOM nodes.
Code Readability
React Fragments improve code readability, by eliminating the use of extraneous wrapper elements or divs. This streamlines codebases and makes them more understandable, which improves maintainability.
Valid HTML
React Fragments are a syntactically and grammatically correct way to return multiple JSX elements. React Fragments replace div elements, which ensures that our HTML is compliant with standards. This reduces the chances of having invalid markup.
Flexible Component Rendering
React Fragments allows the return of multiple JSX components from a component, without the restrictions imposed by a parent element. This flexibility is essential in situations where maintaining a parent-child relationship could otherwise be problematic.
React Fragments are more than just code aesthetics. They’re a performance-boosting technique that follows to the core principles for React development. React. Fragment can help developers achieve cleaner, more readable code, as well as substantial gains in rendering performance.
Now that you have an idea about React Fragment with its syntax and the benefits, you might have the question what is the react fragment vs div, why you select the React Fragments over div.
React Fragments vs <div>: A Comparison
In the dynamic landscape of web development, the selection between utilizing the react framework and relying on traditional HTML div elements represents a fundamental decision that shapes the architecture and efficiency of a web application.
React Frames for Code Cleanliness
Use React Frames to clean up your code and eliminate unnecessary divs from the DOM tree. This choice improves code clean-up and also makes React applications more readable.
Efficiency of Rendering
React Fragments excel in rendering efficiency. They offer a faster response time and less memory consumption than their div counterparts. The streamlined structure makes sure that each element is rendered exactly as intended. This leads to a seamless user experience.
DIV Element DOM Expansion
Although Div elements are familiar, but their excessive use can cause DOM to expand. The large number of nested nodes created by divs can increase memory consumption, and may impact the page loading time. It is important to consider performance when deciding whether to use them.
Memory Consumption
React Fragments boast a more streamlined prototype chain--DocumentFragment -> Node -> EventTarget--
resulting in more memory-efficient rendering. In contrast, div elements carry an expanded prototype chain--HTMLDivElement -> HTMLElement -> Element -> Node -> EventTarget--
introducing additional methods and potentially consuming more memory.
Dynamic Reusability
React Fragments excel at reusability and allow efficient reuse of application components. Div elements are useful when creating specific components, or assigning keys to elements. Your React application’s specific goals and requirements will determine which element you choose.
Ensure Optimal Results
There is no single-size-fits all answer to the React Fragment vs Div Element Debate. By balancing the two according to your application, you can achieve a perfect blend of readability and performance. Use React Fragments to create a simple, clean structure. Reserve divs only for situations that require specific designs or assignments.
Challenges Faced from <div> Usage
Developers need to keep in mind these challenges associated with div usage to ensure a more streamlined, efficient, & meaningful approach to web development. Here are few of the challenges that you may face while over using the div tag.
Semantic Clutter
Overusing the div element can result in non-semantic HTML structures that are cluttered and difficult to read.
SEO and Accessibility Impact
The overuse of divs can hinder SEO and accessibility efforts. When Div’s are heavy coded it may not provide meaningful information for assistive technologies or search engines.
Bloating of the Document Object Model
An excessive reliance on divs can lead to a bloated DOM. This will impact performance and could result in slower page loading times.
Semantic Meaning Lack
Divs are lacking of semantic meaning and make it difficult to maintain an outline that is meaningful for the understanding of the structure of content.
CSS Complications
The use of divs in large quantities can make CSS styling and layout more difficult. This could lead to an increase in complexity and difficulty maintaining a consistent look.
Now that we have covered the challenges, let’s see how you can use the key prop with the React Fragments.
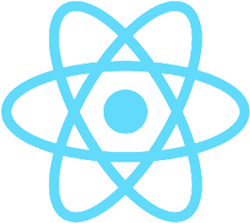
Amplify Your In-House Expertise with
Aglowid’s Seasoned ReactJS Specialists
Using the Key Prop with React Fragments
In certain cases, the keyprop is needed in a React app. Key props in react are used to control component instance. In scenarios such as this, react uses key props to identify the items that have been changed, removed or added. The code below shows how to use key props with fragments in React applications.
function CustomerData () {
return (
{data.map(dat=>
<React.Fragment key={dat.id}>
<td>{dat.name}</td>
</React.Fragment>
)}
);
}
Apart from this, there is also a shorthand notation to wrap multiple elements together. Let’s look at it in detail.
Using Shorthand Notation
React provides not only React Fragment to wrap elements, but also a shorthand <></> that is similar to React Fragment with a smaller memory footprint. The shorthand notation <></> in a react application is implemented by the following.
<function Table One () {
return (
<>
<td>Eat</td>
<td>Train</td>
<td>Sleep</td>
<>
);
}
Okay, this was generated using the short notation, what if you make this in HTML
<table>
<tr>
<div>
<td>Eat</td>
<td>Train</td>
<td>Sleep</td>
</div>
</tr>
</table>
However, there are some drawbacks to this approach. For example, implementing the key prop is impossible here as the shorthand notation <></> wont work here.
Implementation Of Using React Fragments
Let’s understand the implementation of React Fragments with an example.
export default function Blog() {
return (
<>
<Post title="An update" body="Hello! I am updating the blog today" />
<Post title="CodeSandBox" body="Hello! I am CodeSandBox" />
</>
);
}
function Post({ title, body }) {
return (
<>
<PostTitle title={title} />
<PostBody body={body} />
</>
);
}
function PostTitle({ title }) {
return <h1>{title}</h1>;
}
function PostBody({ body }) {
return (
<article>
<p>{body}</p>
</article>
);
}
In the code given above, the separate item component is defined. This item component uses the React Fragment to wrap the name and title elements. Then the same item element is used in the App component to render the list of items.
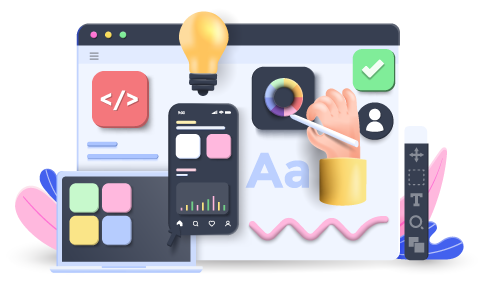
Cutting Costs but
NOT Cutting Quality
that’s how we roll!
Wrapping Up!
This brings us to the end of the React fragment discussion. For a more streamlined, understandable code solution in React apps, React Fragments shines as a flexible option. They enhance the code’s visual appeal and preserve the semantic HTML structure by allowing items to be grouped together without the need for extra div wraps. React Fragments are an easy way to create modular and effective React components. They may be used for anything from rendering lists and tables to performance optimization. When it comes to building scalable and stable user interfaces in compliance with best practices, developers can benefit greatly from them because of their efficiency and flexibility.