Quick Summary:
Android apps are indispensable yet susceptible to cyber threats. This guide centers on Secure Android App Development, addressing risks, and empowering developers to safeguard user’s data and privacy, ensuring the reliability of Android applications.
Mobile applications have become deeply ingrained in modern life, providing convenience, efficiency, and connectivity at our fingertips. Android apps have transformed industries and daily routines by streamlining tasks and offering user-friendly access to powerful functions.
However, the ubiquity of Android apps presents significant security risks, especially, when Android is the favorite target of hackers. Sensitive users’ data including financial information, communications & personal details, are prime targets of hackers.
Let’s see what is the importance of Android Security by looking at the stats below.
According to Now Secure,
- 82% of Android devices face one of 25 OS vulnerabilities
- Business apps are 3x more prone to leaking log-in credentials
- One in four mobile apps contains high-risk security flaws
- 50% of 5-10 million download apps have security flaws
- 25% of 2 million Google Play apps have security flaws
- 35% of communications sent by mobile devices are unencrypted
- More than 1/3rd of the data transmitted by a device is exposed
- Each mobile connects to an average of 160 unique IPs
- 43% of mobile device users need passcode or pattern on their phone
- Social media apps are 3 times more prone to password exposure
- 7% of mobile apps include at least 1 high-risk security flaw
- Half of the apps with 5 to 10 million downloads include the security flaw
What is the importance of Android App Security?
Mobile devices contain a wealth of sensitive user information, from personal photos and messages to banking credentials and identity details.
Android developers must take proper precautions to protect that user data and ward off attacks. This requires a comprehensive approach to security, including encryption, secure coding practices, and using robust Android App Development Libraries for added defense.
Common security risks found in Android apps include:
- Data leakage – User data can be leaked or exposed through insecure connections, improper error handling, logging sensitive info, etc.
- Malware – Malicious apps or code snippets can steal data, encrypt user files for ransom, spy on users, and more.
- Man-in-the-Middle Attacks – Traffic interception allows attackers to view unencrypted data sent between apps and servers.
- Code Tampering – Hackers can decompile apps and reverse engineer code to find vulnerabilities.
Neglecting Android security best practices leaves apps—and users—much more vulnerable to data breaches, privacy violations, and hacks. To mitigate these security risks, it’s important to apply secure coding practices.
Android App Development Security Best Practices
Engaging security best practices during all phases of development is essential to creating Android apps that safeguard user data against emerging threats. By following android app development security best practices, developers can construct the layered defense to protect the app against security threats, and understanding the Android app development cost involved in implementing these security measures is equally crucial for effective planning and protection.
Implement Proper App Permissions
The Android permission system is a core security mechanism for controlling access to protected data and OS features. Apps must request permission to access sensitive user data like contacts, SMS, location, camera, etc.
Here are some tips for handling Android permissions securely:
- Only request the bare minimum permissions needed for your app’s core functionality. Avoid asking for unnecessary or excessive permissions.
- Use runtime permissions for more sensitive permissions that aren’t critical for app functioning. Introduced in Android 6.0, runtime permissions display a prompt to the user in-context when the permission is needed.
- For normal permissions, thoroughly explain why each permission is required during the installation process. Users are more likely to accept permissions they understand.
- Never request signature permission levels solely to prevent other apps from accessing your components. Use proper security measures instead.
- Follow principle of least privilege by separating access between UI and background threads. Only give the minimum permissions needed for each context.
- Handle permission denial gracefully. Your app shouldn’t crash or fail just because a user denied a permission request.
Getting permission design rights goes a long way towards securing user data and limiting attack surfaces.
Secure Handling of App Data
Data traveling between an Android handset and the backend of your server is one of the first things attackers will examine when aiming for Android devices. They can learn a ton about your app by listening in on those exchanges. If you’re unlucky, they could even be able to exploit the information to figure out how to mimic your app and obtain unauthorized access to server-side data.
Therefore, the first step in protecting an Android app is straightforward: use strong encryption to safeguard the data transmission layer. From a security perspective, how you handle app data in this case is crucial. To manage the sensitive data securely, use these android app development security best practices:
Encrypt Local Data Storage
Encrypting local data storage adds a layer of defense in case devices get lost or stolen. Here are some options:
- SQLCipher – Provides 256-bit AES encryption for SQLite databases on Android. Allows secure storage of sensitive info in a database.
- Jetpack Security – Encrypts files, shared preferences and databases with a user-provided secret key. Easy to integrate with Jetpack libraries.
- EncryptedPreferences – Encrypts SharedPreferences with AES 128-bit encryption. It helps protect stored preferences.
Avoid Leaked Data Through Logs
Logging data for debugging purposes can inadvertently expose sensitive user info like passwords, emails, API keys, etc.
- Never log messages containing user credentials or personal data.
- Obfuscate identifiers like usernames before logging.
- In production builds, disable logging entirely or log only generic messages.
Validate and Sanitize Inputs
Validating and sanitizing any user-supplied data helps prevent bugs and security breaches:
- Validate inputs from web APIs, files, databases, and UI forms before use. Check for appropriate type, length, and range.
- Escape dangerous characters when outputting data to prevent XSS, CSRF and other injection attacks.
- Use parameterized queries or stored procedures when passing data to a database. Never build queries by string concatenation.
Use Certificate Pinning
Certificate pinning hardcodes the server certificate into the app and checks it against the certificate provided on each connection. This prevents man-in-the-middle attacks via spoofed certificates.
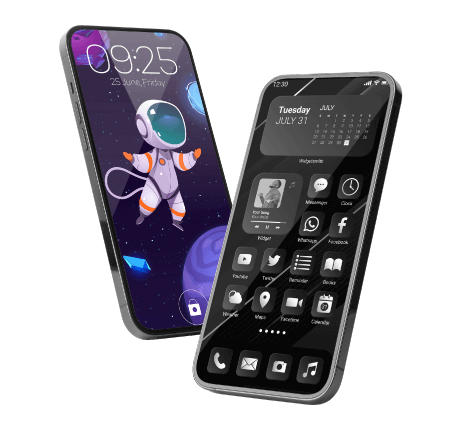
Don’t suffer from ‘app-titude’!📱
Hire Android developers from Aglowid
for ‘app-solutely’
great results!👍
Utilize Impenetrable Authentication Mechanism
The next frequent attack vector after data transfer from an Android device is a weakness in authentication processes. You must do all possible to make sure that authentication is strong and secure. The user’s convenience, however, makes this difficult. But doing so won’t make your software as secure as it can be; instead, you only need to make it as user-friendly as possible.
It is important to use 2FA, OAuth, or OpenID Connect and it is worthwhile to do so. Furthermore, you must be careful about how you handle situations like key swaps. To safeguard the transactions, you should use at the very least AES encryption.
Finally, you must ensure that token-based security is used to verify that the requests coming from your app’s backend are real. As a result, even if a hacker manages to examine a live data stream, they will not be able to use that knowledge to carry out an attack.
Android offers several prebuilt security features for safeguarding apps, including the Android Keystore System and the fingerprint authentication API. To gain a deeper understanding, let’s explore the use of the fingerprint authentication API through an illustrative example.
FingerprintManager fingerprintManager = (FingerprintManager) getSystemService(FINGERPRINT_SERVICE);
fingerprintManager.authenticate(null, null, 0, new AuthenticationCallback(), null);
Implement Regular Updates
Ensuring that your app receives regular updates is among the most important security measures in android app development. Since security risks are continuously changing, keeping up with changes is essential to maintaining the security of your app.
Regular updates not only address vulnerabilities but also enhance your app’s overall security. Additionally, updates might give your users access to fresh features that will keep them interested in and pleased with your program. In order to provide the best possible safety for your users and their data, it is crucial to guarantee that your app is updated on a regular basis.
Utilize Secure Storage
When storing private data on external storage, it’s especially crucial to employ secure storage solutions like Android’s KeyStore. This will assist in ensuring that the data in your app is secured from unauthorized access and that the personal data of your users is kept private.
Secure Network Communication
Apps should use secure protocols and best practices for all network traffic:
Require TLS for All Connections
Encrypt all network traffic with Transport Layer Security (TLS). The benefits include:
- Data is encrypted in transit and safe from interception.
- Mitigates risk of man-in-the-middle attacks.
- Provides server authentication.
Use the latest TLS version whenever possible. Deprecate old SSL versions that have known vulnerabilities.
Validate SSL Certificates
Certificate pinning prevents attackers from using spoofed certificates for man-in-the-middle attacks.
Additionally, always validate SSL certificates from servers and check:
- A trusted CA issues certificate.
- Hostname matches the certificate CN or SAN fields.
- Certificate is not expired and validity dates are sane.
Fail closed and reject connections on invalid certificates.
Avoid Insecure Protocols
Protocols like FTP, telnet, and SMTP transmit data in plaintext. Never use such protocols in mobile apps. Other poor choices include MD5 and SHA1 for secure hashing.
Rigorously Test Application Security
No amount of care in development prevents all possible vulnerabilities. Thoroughly testing app security is crucial:
Dynamic Analysis with Tools
Dynamic analysis through tools like debuggers, malware scanners and fuzzers help uncover flaws:
- Debuggers – Step through app code paths to ensure proper logic and analyze data flow.
- Decompilers – Reverse engineer code to inspect functionality and find potential issues.
- Root detection – Check if rooted/jailbroken devices can bypass protections.
- Fuzz testing – Feed invalid, unexpected random data into apps to trigger crashes and identify flaws.
Conduct Penetration Testing
Hire experts to conduct in-depth penetration testing and attempt real attacks against the app:
- MITM proxies – Intercept traffic between app and server to catch data leaks.
- Reverse engineering – Disassemble and reverse engineer app code to find vulnerabilities.
- Static analysis – Inspect code without executing it to uncover bugs and security issues.
Fix any vulnerabilities uncovered before releasing app updates.
Implement Bug Bounty Programs
Bug bounty programs enable crowdsourced security by incentivizing researchers to disclose vulnerabilities responsibly. Well-designed programs provide rewards to white hat hackers who find and report bugs, helping uncover flaws that may have been missed during in-house testing. Implementing bug bounties strengthens app security through external scrutiny before bugs can be exploited. In other words we can say that, Bug bounty programs allow security researchers to report bugs in return for a reward. This helps discover issues that may have yet to be noticed.
Apply Code Obfuscation
Obfuscating code is an important technique for hindering reverse engineering of Android applications. It transforms and obstructs the original code structure through identifier renaming, control flow modification, and structure manipulation. This process preserves the app’s runtime logic while making the code vastly more challenging to analyze and comprehend. Implementing obfuscation significantly raises the effort required for attackers to understand code functionality and tamper with apps to exploit vulnerabilities.
Practice Input Validation & Sanitization
Input validation and sanitization are critical for securing Android apps against injection attacks and unintended behavior from malicious data. All user-supplied data from sources like web APIs, databases, and UI forms should be validated for appropriate type, length, and range before use. Dangerous characters and syntax should be escaped, encoded, or removed through sanitization routines to prevent code injection, XSS attacks, and other potential exploit vectors targeting the insecure handling of external inputs.
Use Authorized APIs
To protect against unauthorized access & potential attacker misuse, it is vital to use only authorized and properly implemented APIs. APIs that lack robust authorization checks can inadvertently grant elevated privileges to attackers. For example, caching auth tokens locally simplifies API usage for developers but also opens the door for exploitation. To maximize API security, authorization should be handled centrally, not locally. Developers must also validate all input data from custom APIs to prevent issues like code injection that could be used to access features illegally. Following best practices for API authorization, input sanitization and access control is essential.
These are some of the important android app development security best practices that you should implement for secure android app development. To help you with the security aspect there are various popular tools that you can utilize in android development app security. Let’s look at them.
Top Android Security App Development Tools to Use in 2023
With the rising sophistication of cyberthreats, leveraging robust Android security tools throughout the development lifecycle is vital for secure android app development.
Tool | Description |
Debuggers (Android Studio) | Step through code execution to analyze data flows and catch security issues. |
Static Analysis (QARK, SonarQube) | Inspect code without executing to uncover vulnerabilities. |
Decompilers (JD-GUI, CFR) | Reverse engineer apps to simulate attacks by inspecting code. |
Network Proxies (Burp, OWASP ZAP) | Intercept traffic between app and server to test for data leaks. |
Fuzzing Tools (Intruder) | Feed unexpected random data as input to trigger crashes and find flaws. |
Android Lint | Catch potential bugs and security issues within Android Studio. |
Root Detection (SafetyNet) | Check if rooted devices can bypass security protections. |
Cryptography Libraries (Google Tink) | Provide proven secure implementations of crypto primitives. |
Testing Frameworks (Drozer, Xposed, Frida) | Enable dynamic analysis and runtime manipulation for pen testing. |
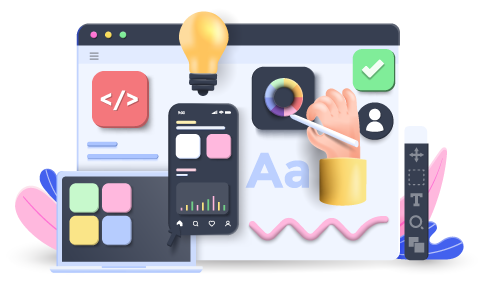
Cutting Costs but
NOT Cutting Quality
that’s how we roll! 🚀
Wrapping up!
As the Android development ecosystem continues to advance, implementing android app development security best practices remains essential. Security measures in android app development like encryption, input validation, penetration testing, and ongoing monitoring help safeguard apps from emerging threats. Additionally, educating users on risks and maintaining compliance builds further trust. Though threats persist, proactive security allows developers to empower people safely through mobile technologies. By making user privacy and safety top priorities, developers can continue innovating while meeting the challenges of our complex, interconnected landscape, which is why it’s crucial to hire an Android app development company that prioritizes security and user safety.